In LINQ, the ToLookup operator is an extension method, and it is useful to extract a set of key/value pairs from the source. Each element in the resultant collection is a generic Lookup object, which holds the key and subsequence items that match the key.
Syntax of LINQ ToLookup Operator
Following is the syntax of using the LINQ ToLookup operator to return collection as a key/value pair.
LINQ ToLookup Syntax in C#
var emp = objEmployee.ToLookup(x => x.Department);
LINQ ToLookup Syntax in VB.NET
Dim emp = objEmployee.ToLookup(Function(x) x.Department)
If you observe above syntax we are converting “objEmployee” collection to key / value pair list using ToLookup() operator.
LINQ ToLookup() Operator in Method Syntax Example
Following is the example of using LINQ ToLookup() in method syntax to convert input collection items as key/value pair lists.
C# Code
using System;
using System.Collections.Generic;
using System.Linq;
namespace Linqtutorials
{
class Program
{
static void Main(string[] args)
{
List<Employee> objEmployee = new List<Employee>()
{
new Employee(){ Name="Ashish Sharma", Department="Marketing", Country="India"},
new Employee(){ Name="John Smith", Department="IT", Country="Australia"},
new Employee(){ Name="Kim Jong", Department="Sales", Country="China"},
new Employee(){ Name="Marcia Adams", Department="HR", Country="USA"},
new Employee(){ Name="John Doe", Department="Operations", Country="Canada"}
};
var emp = objEmployee.ToLookup(x => x.Department);
Console.WriteLine("Grouping Employees by Department");
Console.WriteLine("---------------------------------");
foreach (var KeyValurPair in emp)
{
Console.WriteLine(KeyValurPair.Key);
// Lookup employees by Department
foreach (var item in emp[KeyValurPair.Key])
{
Console.WriteLine("\t" + item.Name + "\t" + item.Department + "\t" + item.Country);
}
}
Console.ReadLine();
}
}
class Employee
{
public string Name { get; set; }
public string Department { get; set; }
public string Country { get; set; }
}
}
VB.NET Code
Module Module1
Sub Main()
Dim objEmployee As New List(Of Employee)() From {
New Employee() With {.Name = "Ashish Sharma", .Department = "Marketing", .Country = "India"},
New Employee() With {.Name = "John Smith", .Department = "IT", .Country = "Australia"},
New Employee() With {.Name = "Kim Jong", .Department = "Sales", .Country = "China"},
New Employee() With {.Name = "Marcia Adams", .Department = "HR", .Country = "USA"},
New Employee() With {.Name = "John Doe", .Department = "Operations", .Country = "Canada"}
}
Dim emp = objEmployee.ToLookup(Function(x) x.Department)
Console.WriteLine("Grouping Employees by Department")
Console.WriteLine("---------------------------------")
For Each KeyValurPair In emp
Console.WriteLine(KeyValurPair.Key)
For Each item In emp(KeyValurPair.Key)
Console.WriteLine(vbTab + item.Name + vbTab + item.Department + vbTab + item.Country)
Next
Next
Console.ReadLine()
End Sub
Class Employee
Public Property Name() As String
Get
Return m_Name
End Get
Set(value As String)
m_Name = Value
End Set
End Property
Private m_Name As String
Public Property Department() As String
Get
Return m_Department
End Get
Set(value As String)
m_Department = Value
End Set
End Property
Private m_Department As String
Public Property Country() As String
Get
Return m_Country
End Get
Set(value As String)
m_Country = Value
End Set
End Property
Private m_Country As String
End Class
End Module
In the above example, we grouped Employees by Department using the ToLookup method. Since ToLookup produces key/value pair, we used it in the foreach loop, and the inner loop extracts the values based on the Key passed as input.
Output LINQ ToLookup() Operator Example
Following is the result of the LINQ ToLookup() operator example to convert collection to key/value pair collection.
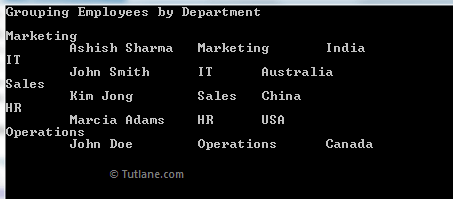
LINQ ToLookup in Query Syntax Example
Following is the example of using ToLookup in query syntax
C# Code
using System;
using System.Collections.Generic;
using System.Linq;
namespace Linqtutorials
{
class Program
{
static void Main(string[] args)
{
List<Employee> objEmployee = new List<Employee>()
{
new Employee(){ Name="Ashish Sharma", Department="Marketing", Country="India"},
new Employee(){ Name="John Smith", Department="IT", Country="Australia"},
new Employee(){ Name="Kim Jong", Department="Sales", Country="China"},
new Employee(){ Name="Marcia Adams", Department="HR", Country="USA"},
new Employee(){ Name="John Doe", Department="Operations", Country="Canada"}
};
var emp = (from employee in objEmployee select employee).ToLookup(x => x.Department);
Console.WriteLine("Grouping Employees by Department");
Console.WriteLine("---------------------------------");
foreach (var KeyValurPair in emp)
{
Console.WriteLine(KeyValurPair.Key);
// Lookup employees by Department
foreach (var item in emp[KeyValurPair.Key])
{
Console.WriteLine("\t" + item.Name + "\t" + item.Department + "\t" + item.Country);
}
}
Console.ReadLine();
}
}
class Employee
{
public string Name { get; set; }
public string Department { get; set; }
public string Country { get; set; }
}
}
VB.NET Code
Module Module1
Sub Main()
Dim objEmployee As New List(Of Employee)() From {
New Employee() With {.Name = "Ashish Sharma", .Department = "Marketing", .Country = "India"},
New Employee() With {.Name = "John Smith", .Department = "IT", .Country = "Australia"},
New Employee() With {.Name = "Kim Jong", .Department = "Sales", .Country = "China"},
New Employee() With {.Name = "Marcia Adams", .Department = "HR", .Country = "USA"},
New Employee() With {.Name = "John Doe", .Department = "Operations", .Country = "Canada"}
}
Dim emp = (From employee In objEmployee).ToLookup(Function(x) x.Department)
Console.WriteLine("Grouping Employees by Department")
Console.WriteLine("---------------------------------")
For Each KeyValurPair In emp
Console.WriteLine(KeyValurPair.Key)
For Each item In emp(KeyValurPair.Key)
Console.WriteLine(vbTab + item.Name + vbTab + item.Department + vbTab + item.Country)
Next
Next
Console.ReadLine()
End Sub
Class Employee
Public Property Name() As String
Get
Return m_Name
End Get
Set(value As String)
m_Name = Value
End Set
End Property
Private m_Name As String
Public Property Department() As String
Get
Return m_Department
End Get
Set(value As String)
m_Department = Value
End Set
End Property
Private m_Department As String
Public Property Country() As String
Get
Return m_Country
End Get
Set(value As String)
m_Country = Value
End Set
End Property
Private m_Country As String
End Class
End Module
Result of LINQ ToLookup() in Query Syntax Example
Following is the result of LINQ ToLookup() in query syntax example.
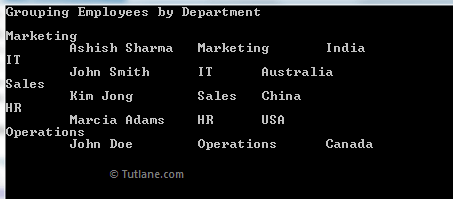
This is how we can use LINQ ToLookup() method in c#, vb.net to extra key/value pair from the given list/collection values with query syntax and method syntax.