LINQ to XML is a programming interface that enables us to write LINQ queries on XML documents to get the required data.
The LINQ to XML will bring the XML document into memory and allow us to write LINQ queries on in-memory XML documents to get XML document elements and attributes.
To use LINQ to XML functionality in our applications, we need to add a “System.Xml.Linq” namespace reference.
Syntax of LINQ to XML
Following is the syntax of writing LINQ queries on XML data.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
var result = from ed in doc.Descendants("employee")
select new
{
Id = ed.Element("empid").Value,
Name = ed.Element("empname").Value
};
VB.NET Code
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
Dim result = From ed In doc.Descendants("employee") Select New With {.Id = ed.Element("empid").Value, .Name = ed.Element("empname").Value}
If you observe above syntax we are reading xml file data and writing LINQ queries to get required data from XML.
LINQ to XML Example
Now we will see how to use XML with LINQ queries in the asp.net web application with example. To create an application, follow the below steps.
Open visual studio à Go to File à Select New à Select Project as shown below.
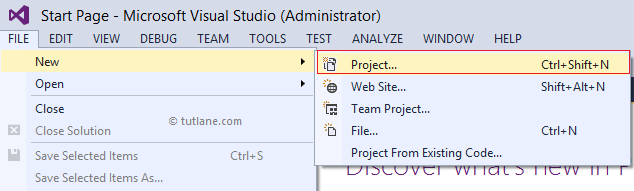
Once we select a project new pop-up will open in that select Asp.Net Empty Web Application then give name as “LINQtoXML” and click an OK button like as shown below.
To work with LINQ and XML, we will add one XML file in our application. For that, right-click on your application à select Add à select New Item as shown below.
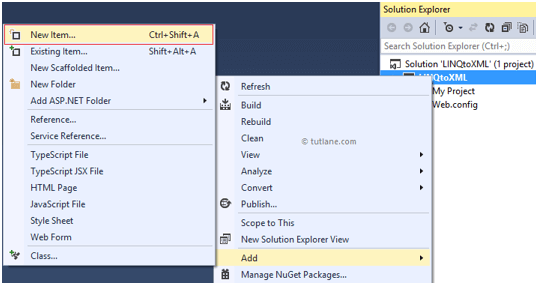
Once we click on New Item new pop-up will open in that select XML file from the Data section à Give a name for that XML file à Click Add button as shown below.
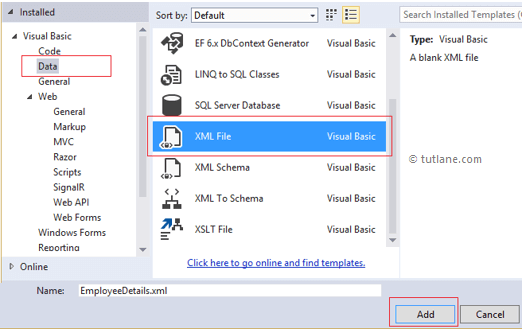
Once we add an XML file, open it and add some records like as shown below.
<?xml version="1.0"encoding="utf-8" ?>
<Employees>
<employee>
<empid>1</empid>
<empname>Suresh Dasari</empname>
<salary>10000</salary>
<gender>Male</gender>
</employee>
<employee>
<empid>2</empid>
<empname>Rohini Alavala</empname>
<salary>20000</salary>
<gender>Female</gender>
</employee>
<employee>
<empid>3</empid>
<empname>Praveen Alavala</empname>
<salary>30000</salary>
<gender>Male</gender>
</employee>
<employee>
<empid>4</empid>
<empname>Sateesh Chandra</empname>
<salary>50000</salary>
<gender>Male</gender>
</employee>
<employee>
<empid>5</empid>
<empname>Sushmitha</empname>
<salary>60000</salary>
<gender>Female</gender>
</employee>
</Employees>
Now we will show EmployeeDetail xml data in our application for that Right-click on application à select Add à New Item à Select Web Form à Give name as Default.aspx and click OK button.
Now open the Default.aspx page and write the code as shown below.
Default.aspx
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Bind Gridview with XML File Data</title>
<style type="text/css">
.GridviewDiv {font-size: 100%; font-family: 'Lucida Grande', 'Lucida Sans Unicode', Verdana, Arial, Helevetica, sans-serif; color: #303933;}
.headerstyle
{
color:#FFFFFF;border-right-color:#abb079;border-bottom-color:#abb079;background-color: #df5015;padding:0.5em 0.5em 0.5em 0.5em;text-align:center;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div class="GridviewDiv">
<asp:GridView ID="gvDetails" runat="server">
<HeaderStyle CssClass="headerstyle" />
</asp:GridView>
</div>
</form>
</body>
</html>
Now open the code behind file and write the following code in page load.
C# Code
using System;
using System.Linq;
using System.Web.UI;
using System.Xml.Linq;
namespace LINQtoXML
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
var result = from ed in doc.Descendants("employee")
where Convert.ToInt32(ed.Element("salary").Value) >= 20000
select new
{
Id = ed.Element("empid").Value,
Name = ed.Element("empname").Value,
Salary = ed.Element("salary").Value,
Gender = ed.Element("gender").Value
};
gvDetails.DataSource = result;
gvDetails.DataBind();
}
}
}
}
VB.NET Code
Imports System.Linq
Imports System.Web.UI
Imports System.Xml.Linq
Public Class WebForm1
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Page.IsPostBack Then
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
Dim result = From ed In doc.Descendants("employee") Where
Convert.ToInt32(ed.Element("salary").Value) >= 20000 Select New With {.Id = ed.Element("empid").Value, .Name = ed.Element("empname").Value, .Salary = ed.Element("salary").Value, .Gender = ed.Element("gender").Value}
gvDetails.DataSource = result
gvDetails.DataBind()
End If
End Sub
End Class
If you observe the above example, we added namespaces like “System.Xml.Linq” it will allow us to read xml document data, and we write conditions on XML data to get records where Salary is greater than equal to 20000. Now we will run and see the result of the application.
Result of LINQ to XML Example
Following is the result of the LINQ to XML example.
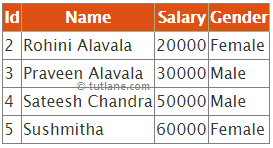
We will learn some more code snippets in LINQ to XML to get the required data from XML documents.
LINQ to XML Add New Element
Following is the code snippet to add new elements to the XML file dynamically using LINQ to XML.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
doc.Add(new XElement("employee",
new XElement("empid", 6),
new XElement("empname", "Madhav Sai"),
new XElement("salary", 80000),
new XElement("gender", "Male")));
VB.NET Code
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
doc.Add(New XElement("employee",
New XElement("empid", 6),
New XElement("empname", "Madhav Sai"),
New XElement("salary", 80000),
New XElement("gender", "Male")))
If you observe the above code snippet, we are adding new elements to the XML file dynamically using LINQ to XML.
LINQ to XML Add Element as First Child of XML File
Following is the syntax of adding new elements as the first child of XML file using LINQ to XML.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
doc.AddFirst(new XElement("employee",
new XElement("empid", 7),
new XElement("empname", "Honey"),
new XElement("salary", 90000),
new XElement("gender", "Female")));
VB.NET Code
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
doc.AddFirst(New XElement("employee",
New XElement("empid", 6),
New XElement("empname", "Madhav Sai"),
New XElement("salary", 80000),
New XElement("gender", "Male")))
If you observe the above code snippet, it will add an element at the first position of an XML file.
LINQ to XML Remove Element in XML File
Following is the syntax of removing an element in the XML file using LINQ to XML.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
doc.Descendants("employee").Elements("empname").Where(x => x.Value == "Sushmitha").Remove();
VB.NET Code
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
doc.Descendants("employee").Elements("empname").Where(Function(x) x.Value = "Sushmitha").Remove()
If you observe above code snippet we are deleting element in xml file based on “empname”.
Remove 'n' Number of Elements using LINQ to XML
Following is the syntax of removing one or two or three elements from the XML file using LINQ to XML based on our requirement.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
doc.Descendants("employee").Take(2).Remove();
VB.NET Code
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
doc.Descendants("employee").Take(2).Remove()
If you observe the above syntax, we are deleting the first “2” elements from the XML file using LINQ.
Save / Persist XML Changes using LINQ to XML
We made many changes to an XML file using different methods, but nothing affected the XML file because those changes were in memory, not changed in the XML file. If we want to make our changes reflected in the XML file, we need to call Save() method at the end of our modifications, only our changes will reflect in the XML file.
C# Code
XElement doc = XElement.Load(Server.MapPath("EmployeeDetails.xml"));
doc.Add(new XElement("employee",
new XElement("empid", 6),
new XElement("empname", "Madhav Sai"),
new XElement("salary", 90000),
new XElement("gender", "Male")));
doc.Save(Server.MapPath("EmployeeDetails.xml"));
VB.NET
Dim doc As XElement = XElement.Load(Server.MapPath("EmployeeDetails.xml"))
doc.Add(New XElement("employee",
New XElement("empid", 6),
New XElement("empname", "Madhav Sai"),
New XElement("salary", 90000),
New XElement("gender", "Male")))
doc.Save(Server.MapPath("EmployeeDetails.xml"))
If you observe the above code snippet, we are adding new elements to an XML file, and to persist new changes, we are using the Save method.