In LINQ to SQL, we don't have a LIKE operator, but by using contains(), startswith(), and endswith() methods, we can implement LIKE operator functionality in LINQ to SQL.
The following table shows more details regarding operators we used to achieve LINQ to SQL Like operators.
Operator | Description |
Contains() |
It is equivalent to '%string%' |
StartsWith() |
It is equivalent to 'string%' |
EndsWith() |
It is equivalent to '%string' |
Before we start implementing LINQ to SQL LIKE operator examples, first, create a database with required tables and map those tables to LINQ to SQL file (.dbml). If you don't know the process, check this link create and map database tables to LINQ to SQL file (.dbml).
Once we create and map required tables to the .dbml file now, we will show data in our application for that Right-click on application à select Add à New Item à Select Web Form à Give name as Default.aspx and click OK button.
Now open the Default.aspx page and write the code as shown below.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Show Employee Details in Gridview</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="gvDetails" runat="server"></asp:GridView>
</div>
</form>
</body>
</html>
Now open the code behind file and write the code like as shown below
LINQ to SQL Contains() Operator
In LINQ to SQL, using the Contains() operator, we can get records or rows containing the defined text.
C# Code
protected void Page_Load(object sender, EventArgs e)
{
EmployeeDBDataContext db = new EmployeeDBDataContext();
if (!Page.IsPostBack)
{
var result = from ed in db.EmployeeDetails where ed.EmpName.Contains("su")
select new
{
Name = ed.EmpName,
Location = ed.Location,
Gender = ed.Gender
};
gvDetails.DataSource = result;
gvDetails.DataBind();
}
}
VB.NET Code
Public Class WebForm1
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim db As New EmployeeDBDataContext()
If Not Page.IsPostBack Then
Dim result = From ed In db.EmployeeDetails Where ed.EmpName.Contains("su") Select New With {.Name = ed.EmpName, .Location = ed.Location, .Gender = ed.Gender}
gvDetails.DataSource = result
gvDetails.DataBind()
End If
End Sub
End Class
If you observe the above example, we are trying to get records from the “EmployeeDetails” table where it contains the “su” word.
Result of LINQ to SQL Contains Example
Following is the result of LINQ to SQL Contains operator example.
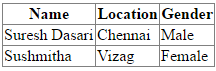
LINQ to SQL StartsWith() Operator
In LINQ to SQL StartsWith() operator is used to get records or rows from the table where records start with the defined word.
C# Code
protected void Page_Load(object sender, EventArgs e)
{
EmployeeDBDataContext db = new EmployeeDBDataContext();
if (!Page.IsPostBack)
{
var result = from ed in db.EmployeeDetails where ed.EmpName.StartsWith("s")
select new
{
Name = ed.EmpName,
Location = ed.Location,
Gender = ed.Gender
};
gvDetails.DataSource = result;
gvDetails.DataBind();
}
}
VB.NET Code
Public Class WebForm1
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim db As New EmployeeDBDataContext()
If Not Page.IsPostBack Then
Dim result = From ed In db.EmployeeDetails Where ed.EmpName.StartsWith("s") Select New With {.Name = ed.EmpName, .Location = ed.Location, .Gender = ed.Gender}
gvDetails.DataSource = result
gvDetails.DataBind()
End If
End Sub
End Class
If you observe an example, we are getting records from the "EmployeeDetails" table where the name starts with "s".
Result of LINQ to SQL StartsWith() Example
Following is the result of LINQ to SQL StartsWith() example.
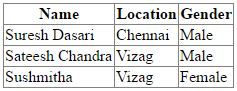
LINQ to SQL EndsWith() Operator
In LINQ to SQL, EndsWith(() operator is useful to return records or rows from the table where records end with a defined word.
C# Code
protected void Page_Load(object sender, EventArgs e)
{
EmployeeDBDataContext db = new EmployeeDBDataContext();
if (!Page.IsPostBack)
{
var result = from ed in db.EmployeeDetails where ed.EmpName.EndsWith("la")
select new
{
Name = ed.EmpName,
Location = ed.Location,
Gender = ed.Gender
};
gvDetails.DataSource = result;
gvDetails.DataBind();
}
}
VB.NET Code
Public Class WebForm1
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim db As New EmployeeDBDataContext()
If Not Page.IsPostBack Then
Dim result = From ed In db.EmployeeDetails Where ed.EmpName.EndsWith("la") Select New With {.Name = ed.EmpName, .Location = ed.Location, .Gender = ed.Gender}
gvDetails.DataSource = result
gvDetails.DataBind()
End If
End Sub
End Class
If you observe the above example, we are getting records from the “EmployeeDetails” table where the name ends with “la”.
Result of LINQ EndsWith() Example
Following is the result of the LINQ EndsWith() example.
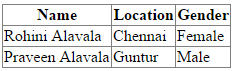
This is how we can achieve Like functionality in LINQ to SQL using Contains(), Startswith(), and Endswith() operations in c#, vb.net.